Table Of Content
Using the SwitchOff method, we can turn Off the Sony TV, and using the SetChannel method, we can change the channel number of the Sony TV. Create a class file named SamsungLedTv.cs and copy and paste the following code. This class implements the LEDTV interface and provides implementations for SwitchOn, SwitchOff, and SetChannel methods. Using the SwitchOff method, we can turn Off the Samsung TV, and with the SetChannel method, we can change the channel number of the Samsung TV.
Coupling avoidance between abstraction and implementation
For all its 21st century complexity, the side-spar design is based on suspension principles first used some two centuries earlier. A Bridge Pattern says that just "decouple the functional abstraction from the implementation so that the two can vary independently". This article taught you about the Bridge design pattern, real-world examples, and its practical implementation.
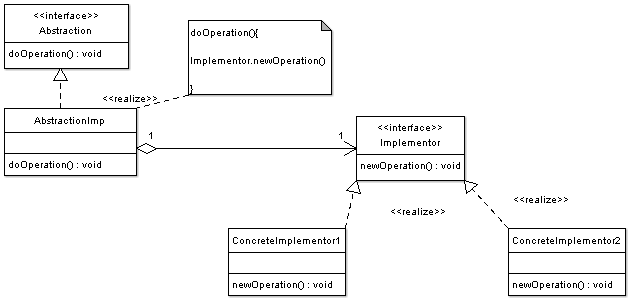
Spring Framework Guru
If there is a specific design pattern that you would like to learn about then let me know so I can provide it for you in the future. Buy the eBook Dive Into Design Patterns and get the access to archive with dozens of detailed examples that can be opened right in your IDE. Now let’s say you want to add one more capability (i.e. delete) at the abstraction layer. It must not force a change in existing implementers and clients as well. You can prevent the explosion of a class hierarchy by transforming it into several related hierarchies.
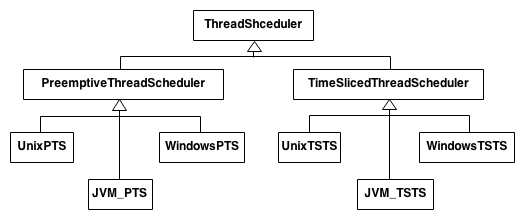
Introducing Bridge Pattern
The Bridge Design Pattern helps to avoid the need for multiple inheritance, which can lead to complex and hard-to-maintain code. Bridge is used when we need to decouple an abstraction from its implementation so that the two can vary independently. This type of design pattern comes under structural pattern as this pattern decouples implementation class and abstract class by providing a bridge structure between them. We use abstraction to decouple client code from implementations, and the usual way is to use inheritance. We define an interface or an abstract class and create inheritance hierarchies from it, one for each of the several possible implementations. Although at first look this approach appears logical and nothing wrong in it, abstractions through inheritance isn’t always flexible.
Free Intro to Spring Boot Online Course
This gives you the ability to keep the Aimpl header private, and reduce the compile time. Connect and share knowledge within a single location that is structured and easy to search. On the other side of the bridge, we have the Resource class which also contains two methods snippet() and url(), both of which are overridden in the Concrete Implementation (Here, Artist class). Let's look at the structure (UML diagram) of the Bridge Design Pattern and understand how it can be used to solve the above-discussed issue.
Structural Design Patterns
You can solve the above problem by decoupling the Vehicle and Workshop interfaces in the below manner. If you want to change the Bus class, then you may end up changing ProduceBus and AssembleBus as well and if the change is workshop specific then you may need to change the Bike class as well. Regardless of the structure, every bridge must stand strong under the two important forces we'll talk about next.
Share the implementation among multiple clients
When using subclassing, different subclasses implement an abstract class in different ways. But an implementation is bound to the abstraction at compile-time and cannot be changed at run-time. The bridge pattern is often confused with the adapter pattern, and is often implemented using the object adapter pattern; e.g., in the Java code below. Abstraction (also called interface) is a high-level control layer for some entity. It should delegate the work to the implementation layer (also called platform). Longer bridges are created by placing single spans next to each other, with modern beam bridges usually built from a combination of steel and reinforced concrete.
5 best Minecraft bridge ideas and designs in 2022 - Sportskeeda
5 best Minecraft bridge ideas and designs in 2022.
Posted: Fri, 25 Mar 2022 07:00:00 GMT [source]
As a result, you can change the GUI classes without touching the API-related classes. Moreover, adding support for another operating system only requires creating a subclass in the implementation hierarchy. The previous version of the software called “DailyJournal” is not discarded yet, and the users can also access the pages on the previous version.
Similar Reads
For instance, a case where an employee class object is shared among various other classes of an employee management system to implement the complete business logic of the software. The implementation of bridge design pattern follows the notion to prefer Composition over inheritance. The abstraction contains a reference to the implementation object, therefore it can control its methods.
The Bridge design pattern is used to decouple an abstraction from its implementation. As a result, the abstraction and implementation can be developed and changed independently. This pattern is often used to enable the separation of concerns.
You should use the Bridge Design Pattern when you want to separate the abstraction and its implementation so that they can evolve independently. This pattern is useful when you have multiple implementations of the same interface or when you want to avoid the complexity of multiple inheritance. It can also be useful when you want to change the implementation of an abstraction at runtime. The Bridge pattern attempts to solve this problem by switching from inheritance to the object composition.
This is a design mechanism thatencapsulates an implementation class inside of an interface class. The bridge pattern addresses all such issues by separating the abstraction and implementation into two class hierarchies. This figure shows the design without and with the bridge pattern. Let’s say you have a bunch of Shape classes called Circle and Square. This means that you would end up with four classes like GreenSquare and RedCircle. For example, our java classes without bridge design pattern look like below.
We are demonstrating use of Bridge pattern via following example in which a circle can be drawn in different colors using same abstract class method but different bridge implementer classes. As discussed earlier, we use the Bridge Pattern whenever we need to avoid the inheritance-related binding between the abstraction and implementation. This decoupling helps us to separate the abstraction from the implementation allowing us to develop both the abstraction and the implementation independently of each other.
If we make any changes in the Implementation Layer, it won’t affect the Abstraction Layer. Similarly, if we make any changes in the Abstraction Layer, it won’t affect the Implementation layer. In this article, I will discuss the Bridge Design Pattern in C# with Examples.
This pattern involves an interface which acts as a bridge which makes the functionality of concrete classes independent from interface implementer classes. Both types of classes can be altered structurally without affecting each other. Whereas the Bridge pattern is used for code that is more likely to be greenfield. You're designing the Bridge to provide an abstract interface for an implementation that needs to vary, but you also define the interface of those implementation classes. The Bridge pattern is an application of the old advice, "prefer composition over inheritance".It becomes handy when you must subclass different times in ways that are orthogonal with one another. You wouldn't subclass Shape with Rectangle and Circle and then subclass Rectangle with RedRectangle, BlueRectangle and GreenRectangle and the same for Circle, would you?
No comments:
Post a Comment